Element-wise Operators in Python
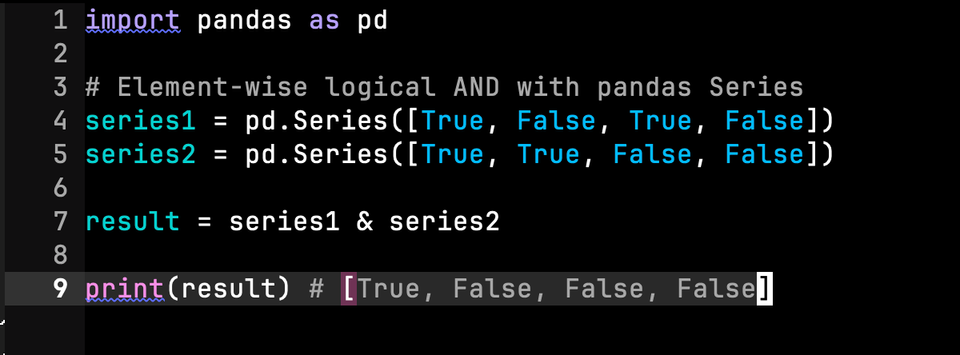
Python is like a trusty sidekick in my coding adventures – a dependable ally when I dive into the world of data-intensive APIs or data analysis. Though Python isn’t my primary language, I've built applications and numerous Jupyter notebooks filled with data analysis and machine learning algorithms. My journey with Python isn’t constant, but it's always enlightening.
One Python feature that often trips me up involves compound conditionals within a DataFrame. It's like a familiar stumbling block I encounter, leading me to Google for solutions. This time, I paused for a deeper understanding, particularly around using a single &
in DataFrame conditionals. It was a moment of self-reflection on my coding knowledge.
The Code
Consider this piece of code:
df[(df["some column"] == "some value") & (df["some other column"] == "some other value")]
The Mistakes
Here's where I usually slip:
Using two **&
**s instead of one:
df[(df["some column"] == "some value") && (df["some other column"] == "some other value")]
Forgetting the parentheses:
df[df["some column"] == "some value" & df["some other column"] == "some other value"]
These missteps stem from my experience with other programming languages, where &&
is common for joining conditions. In Python, and
is used in if/else
statements, a concept I mistakenly linked to DataFrame conditionals. This mental model led me close, but not quite to the reality of pandas DataFrame.
The second error, skipping parentheses, also traces back to my mental model. With if/else
statements, comparison operators take precedence over and
or &&
. This means the individual statements on either side of and
are evaluated first.
So, why the confusion? Mostly, my experience with bitwise operations is minimal. They've been a tiny fraction of my coding work. I've learned about them but haven't used them extensively, leaving a gap in my understanding. And why does bitwise matter here?
In my quest to understand my mistakes with DataFrame conditionals, I discovered that the single &
in pandas is an overloaded bitwise operator, adapted for DataFrame and numpy.
I might not have encountered these issues if I had linked &
with Python's bitwise operations, rather than the and
keyword used in if/else
statements. Notably, not all languages prioritize the bitwise &
operator the same way Python does. This was a key piece of the puzzle for me.
Some coding habits could have prevented these errors, despite my incorrect mental model:
- Routinely using parentheses to group conditions.
- Avoiding compound conditions by writing two statements
Both practices likely lead to cleaner code. I even peeked at the Google Python Style Guide for any recommendations on these practices but didn't find specific guidance.
The Learnings
How It Works
The bitwise &
deals with the binary representation of integers. It converts integers to binary and compares each bit. If both bits are 1, the resulting integer gets a 1 in that place.
# Bitwise AND of integers
a = 12 # In binary: 1100
b = 6 # In binary: 0110
result = a & b # Binary: 1100 & 0110 = 0100 (4 in decimal)
print(result) # Output: 4
For array-like objects, such as lists or pandas Series, the bitwise &
is modified to perform element-wise operations.
import pandas as pd
# Element-wise logical AND with pandas Series
series1 = pd.Series([True, False, True, False])
series2 = pd.Series([True, True, False, False])
result = series1 & series2
print(result) # Output: [True, False, False, False]
Python Order of Operator Precedence
Understanding the order in which Python evaluates different operators was a game changer:
- Parentheses
()
: Used to override the default precedence, so expressions inside parentheses are evaluated first. - Exponentiation
*
: Right-to-left associativity. - Unary Plus
+
, Unary Minus ``, Bitwise NOT~
: These unary operators have the same level of precedence. - Multiplication ``, Division
/
, Floor Division//
, Modulus%
: Left-to-right associativity. - Addition
+
, Subtraction ``: Left-to-right associativity. - Bitwise Shifts
<<
,>>
: Left-to-right associativity. - Bitwise AND
&
: Left-to-right associativity. - Bitwise XOR
^
: Left-to-right associativity. - Bitwise OR
|
: Left-to-right associativity. - Comparison Operators
==
,!=
,<
,<=
,>
,>=
,is
,is not
,in
,not in
: These operators all have the same level of precedence and are evaluated left-to-right. - Boolean NOT
not
: Right-to-left associativity. - Boolean AND
and
: Left-to-right associativity. - Boolean OR
or
: Left-to-right associativity. - Conditional Expression
if``else
: Right-to-left associativity. - Lambda Expression
lambda
: Right-to-left associativity. - Assignment Operators
=
,+=
,=
,=
,/=
,//=
,%=
,*=
,&=
,|=
,^=
,>>=
,<<=
: Right-to-left associativity.
Grasping the nuances of the element-wise operator and operator precedence reshaped my mental models, clearing up my understanding. I'm now more confident in writing compound conditionals and feel equipped to write cleaner code.
Are you taking time to revisit and refine your understanding as you code? It’s a practice that pays off!